Setting up your environment
1.Installing Python
In order to start our journey to being a pro Python coder, we are going to need to install Python. If your operating system does not provide you with a Python package, you can download an installer from the Python official website
To make sure your Python installation is functional, you can open a terminal window and type python3, or if that does not work, just python. Here is what you should expect to see:
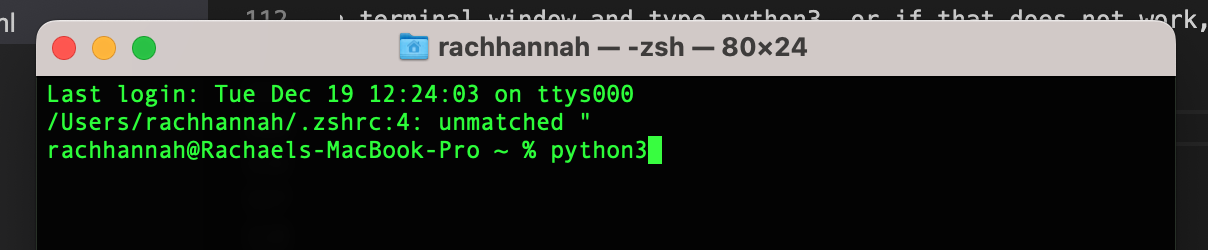
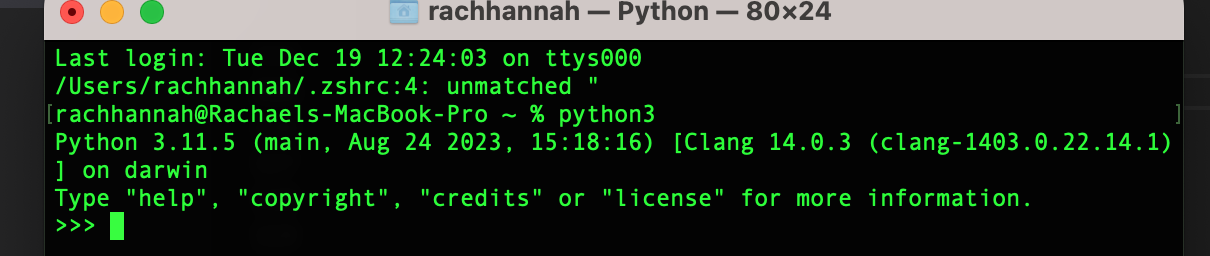
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to computer programmers for software development.
In this tutorial we will use our IDE as source code editor, where we will write and edit our code. If you don't have an IDE installed you can go ahead and install Visual Studio Code
Create a Python File
First you need to create a new folder to store your projects in. Once you've done this open Vistual Studio Code and Go to File > Open and select your folder!
Now lets create a new file and call it something with .py on the end, let's call it hello_world.py:
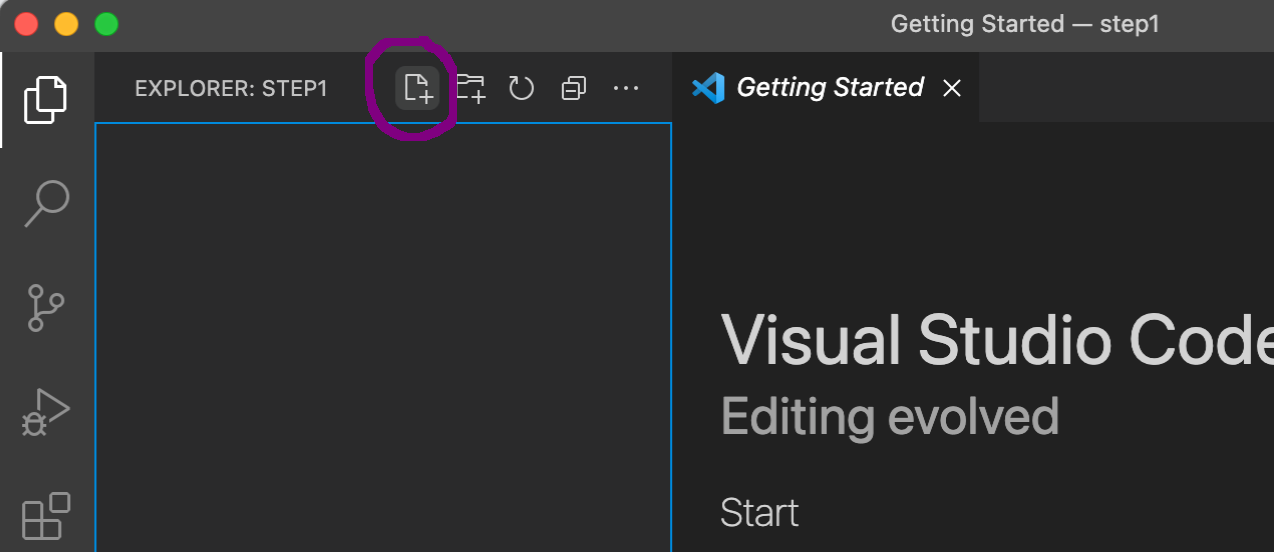

In hello_world.py type:
print("Hello, World!")
3.Run Your Python File
Right-click in the text editor and select "Run Python File in Terminal".
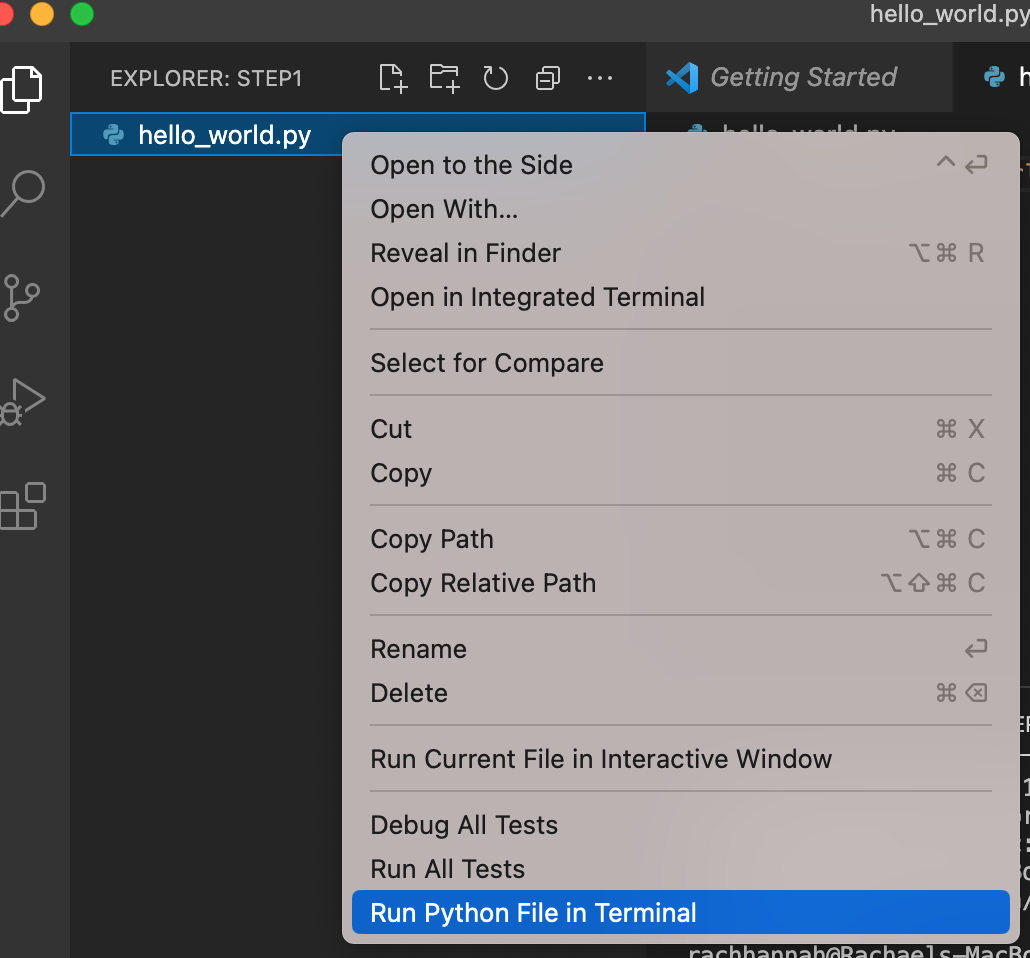
You should see "Hello, World!" printed in the integrated terminal.
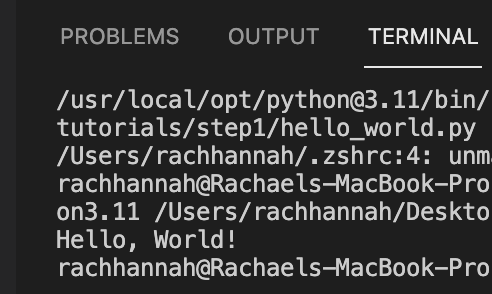
WOHOOO!!!
And there we have it, it's as simple as that.. you've wrote your first line of code!!!!Basics of Python
Now you've succeeeded in writing your first line of code.. Let's go through some basic concepts and exercises. You can type the following exercises in new .py
files and run them in VS code.
1. Comments
Comments in programming are notes that you, the programmer, can add to your code to describe what it does. These comments are not executed as part of the program; their sole purpose is to be read by humans. This is crucial for making your code understandable to others, including your future self. In Python, and many other programming languages, there are specific ways to write comments.
Single-line CommentsIn Python, you create a single-line comment by using the hash symbol #. Everything to the right of the # on that line will be ignored by the Python interpreter.
# This is a single-line comment in Python.
print("Hello, World!") # This prints a greeting.
Here, # This is a single-line comment in Python. is a comment, and it doesn't affect how your code runs. The second comment, # This prints a greeting., is on the same line as some code, but it's still ignored by the interpreter.
Let's give this a try in our hello_world.py file.
2. Variables and Data Types:
Variables:
Think of a variable as a storage box where you can keep something for later use. In coding, a variable is a name that refers to a place in the program memory where you can store data and retrieve it using the variable name.
For example, if you have a piece of information like your name that you want to remember for later use in your program, you can store it in a variable.
name = "Alex"
Data Types:
Data types are simply the type of data that can be stored in a variable. There are several basic data types in Python:
- String: For text or characters. Strings are written inside quotes.
greeting = "Hello, world!"
- Integer: For whole numbers without a decimal point.
age = 32
- Float: For numbers that require a decimal point.
height = 5.10
- Boolean: For values that can only be True or False.
is_hungry = True
- List: A collection of items in a particular order. Lists can contain items of any data type, and they are written inside square brackets.
colours = ["red", "green", "blue"]
- Dictionary: A collection of key-value pairs. You can look up a value using its key, much like a real dictionary.
contact_info = {"name": "Rach", "email": "[email protected]"}
Your Task
Create a .py file that asigns variables to store each of the above data types and print them.
Try having a play around with the values that you are assigning to the variables!
If you'd like a peek at an example answer click here
If you have something that looks similar to the example above then WOHOO Well done! Gold Stars all around!
3. Lists
A list in Python is like a shopping list or a to-do list. It's an ordered collection of items that can be changed after you create it (in technical terms, it's mutable).
You can put anything in a list: numbers, strings, or even other lists. You write a list by placing all the items (elements) inside square brackets [], separated by commas.
Lets use our example list from above:
colours = ["red", "green", "blue"]
In this list of colours, we have three items, each a string representing a colour.
You can access items in a list by referring to their position, or index, within the list. Note that in Python, list indices start at 0, not 1.
So, colours[0]
would give you 'red'.
Your Task!
Before starting create a new file called something like lists.py

You can see an example here, I have hidden this so you can give it ago yourself before reavealing the answer!
You can see an example here, again I have hidden this so you can give it ago yourself before reavealing the answer!
4. Loops
A loop in Python allows you to go through each item in a list (or any collection such as a dictionary that we saw above) and do something with each item. There are mainly two types of loops in Python: for loops and while loops.
Imagine you have a list of chores to do. You go through each item on the list and do it. Once you've reached the end of the list, you stop. That's what a for loop does in Python.
Here is an example of how you can do this using our example list from above:
for colour in colours:
print(colour)
A while loop, on the other hand, will continue to do something as long as a certain condition is true. For example, "While I am hungry, I will keep eating." In programming, it's similar:
Here's an example of how we do this
number = 0
while number < 5:
print(number)
number = number + 1
Your Tasks!!
Now we've gone through that, let's give it a go!
- 1. Iterate over a list of numbers and print each number multiplied by 2.
- 2. Use a while loop to simulate a countdown from 10 to 0.
Lets walk through these tasks together!
Task 1!
- 1. Create a list of numbers, for example: numbers = [1, 2, 3, 4, 5].
If you need a hint click here.
- 2. Use a for loop to iterate over the list.
- 3. Inside the loop print the result, and run your code!
Before starting create a new file called something like loops.py
If you need a hint click here.
If you need a hint click here.
And just like that, task 1 is done! Now lets move onto the second and final task in this part of the tutorial
Task 2!
Before starting create a new file called something like while_loops.py
- Create a variable countdown and set it to 10.
If you need a hint click here.
- Write a while loop that runs as long as countdown is greater than or equal to 0.
If you need a hint click here.
- Inside the loop, print the current value of countdown.
If you need a hint click here.
- Decrement countdown by 1 at the end of each loop iteration... and run your code!!
If you need a hint click here.
- Optional** When the loop is finished, print "Liftoff!".
If you need a hint click here.
And that wraps up Part 1 of the Basics of Python!! In conclusion we went through, Variable and Data types, we demonstrated the use of Loops and Lists and you Wrote a chunk of code!!
You can move on to part 2 now or come back and complete it another day! I'd LOVE to hear your feedback, or chat about how you got on.